Quickstart
To start receiving webhook events in your app, create and register a webhook endpoint by following these steps:
- Create a webhook endpoint handler to receive event data POST requests.
- Register your endpoint with Tokenz using the Tokenz Dashboard.
- Secure your webhook endpoint.
- Test your webhook endpoint using test payments.
1. Create a handler
Set up an HTTPS endpoint function capable of accepting webhook requests via the POST method. You can utilize Webhook.site to view the payload and test delivery.
Ensure that your endpoint function:
- Handles POST requests with a JSON payload consisting of an event object.
- Quickly returns a successful status code (2xx) before executing any complex logic that might cause a timeout.
2. Register your endpoint
Register your webhook endpoints on the Tokenz Dashboard.
- Go to your
Home
page. - Click
Create new webhook
. - Fill out your URL and an optional description, and select the events you want to receive. We recommend selecting
order_succeeded
at minimum to ensure you never miss a successful customer order. - Click
Create webhook
. - Copy and save the returned signing secret. You will need this to verify the webhooks you received. For security reasons, we only show the secret once.
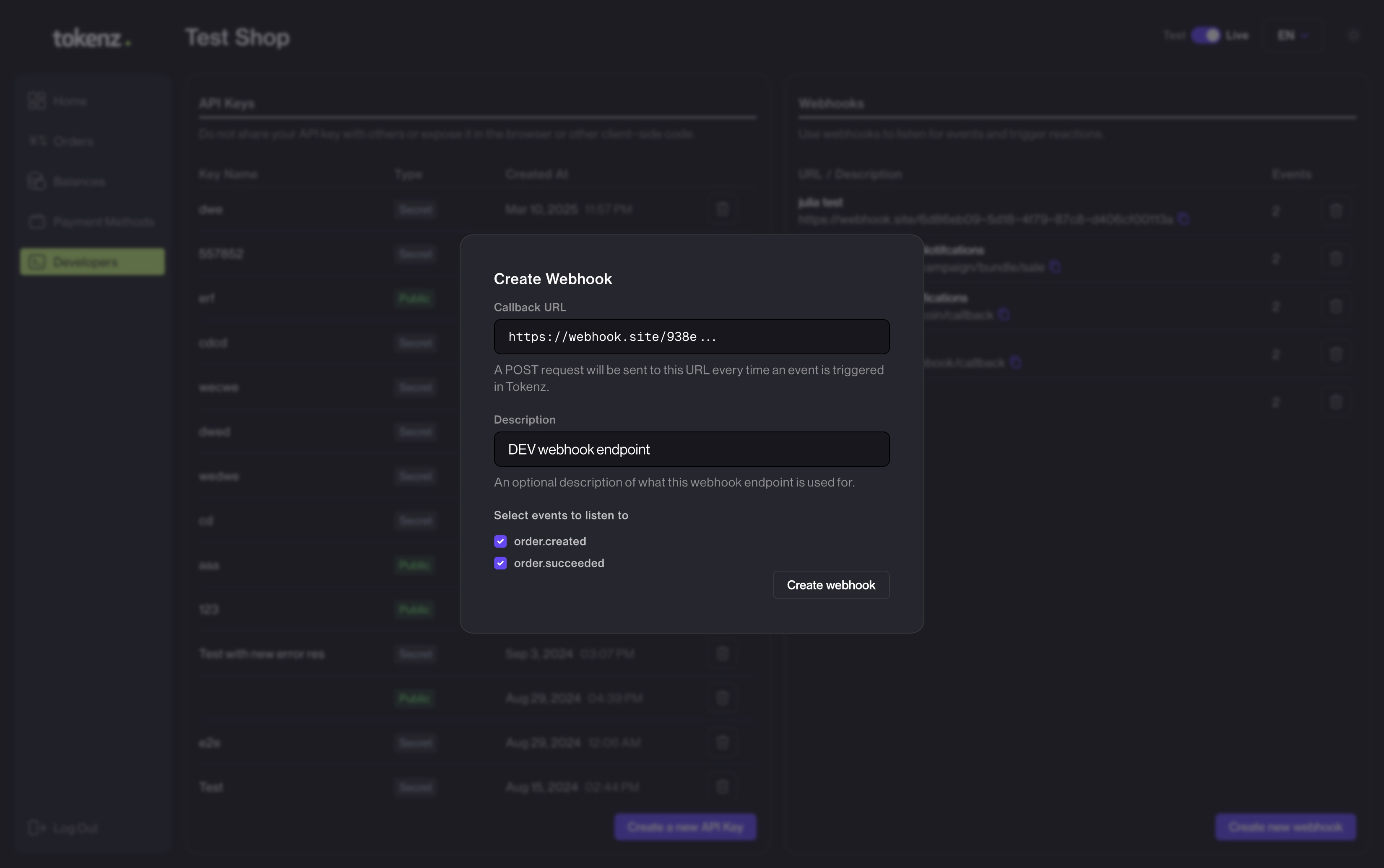
3. Secure your webhook endpoint
Tokenz includes a signature for all webhooks it sends. You must verify this signature to ensure that the webhook is sent by Tokenz and has not been altered. Alternatively, use the webhook notification simply as a trigger to check the order status changes via the API. We recommend verifying the webhook signature to avoid unnecessary API calls.
Each signed event includes a Tokenz-Signature
header containing a timestamp (t:<unix-timestamp>
) and one or more versioned signatures (v1:<HMAC signature>
) needing verification. Currently, the valid live signature scheme is v1
.
Example:
t:1725864981111,v1:zjdrra3...Mh+e6s=
Verifying the Signature
1. Extract the Timestamp and Signatures
Split the header using the comma (,
) as a separator to create a list of elements. Then split each element using the colon (:
) as a separator to derive prefix-value pairs. The prefix t
represents the timestamp, while v1
contains the signature(s). Disregard any other elements.
2. Prepare the Payload for Signing
Formulate the payload string by concatenating:
- The timestamp (as a string)
- The request body verbatim (i.e., the JSON payload)
3. Calculate the Signature
Compute an HMAC using the SHA256 hash function. Use the endpoint's signing secret as the key with the payload string as the message.
4. Compare the Signatures
Match the signature(s) in the header with the expected signature. Evaluate the difference between current and received timestamps to prevent replay attacks. If the signature is valid but the timestamp is outdated, applications can reject the payload. The recommended tolerance is 300 seconds (5 minutes).
A replay attack occurs when a legitimate payload and its signature are intercepted and re-transmitted by an attacker. Tokenz mitigates this by including a timestamp in the Tokenz-Signature
header.
const crypto = require('crypto');
const app = require('express')();
const port = 3000;
app.post("/webhook", (req, res) => {
const secret = 'webhook-endpoint-secret';
const sig = req.headers['tokenz-signature'];
let timestamp, signature;
for (let sigPart of sig.split(',')) {
let [key, value] = sigPart.split(':');
if (key === 't') {
timestamp = value;
} else if (key === 'v1') {
signature = value;
}
}
const checkSignature = crypto.createHmac('sha256', Buffer.from(secret, 'base64'))
.update(`${timestamp}${JSON.stringify(req.body)}`)
.digest('base64');
const currentTimestamp = Math.round(new Date().getTime() / 1000);
if (signature === checkSignature && Math.abs(currentTimestamp - timestamp) <= 300) {
return res.status(200).end();
} else {
return res.status(400).end();
}
});
4. Test your webhook endpoint
To ensure your webhook handling is functioning correctly, create and complete some test payments following our test guide.