Testing
Simulate payments to test your integration.
Introduction
To confirm that your integration works correctly, simulate transactions without moving money. You can do this by creating and manipulating resources in test mode
(use test API keys in all API calls). You can use dedicated test payment methods to complete test checkout sessions.
All test payment checkouts include a test banner at the top to help you quickly identify them.
The test payment methods let you simulate multiple scenarios:
- Successful payment
- Payment errors due to declines or invalid data
- Delayed payment due to async payment methods like bank transfer or convenience store payment
The checkout outcome can be controlled via the Test Authorization Result switch, which is included for testing only.
- Success (成功) will always result in a successful payment
- Synchronous payment methods (cards, PayPay, etc.): You will receive an instant success response.
- Asynchronous payment methods (konbini, pay easy): You will receive a successful response after a 10-second delay.
- Decline (拒否) will never result in a successful payment
- Synchronous payment methods (cards, PayPay, etc.): The payment will be declined, and the checkout will show the payment details input screen to try again (e.g. using a different card).
- Asynchronous payment methods (konbini, pay easy): The payment will never be completed, and the order will stay in processing status.
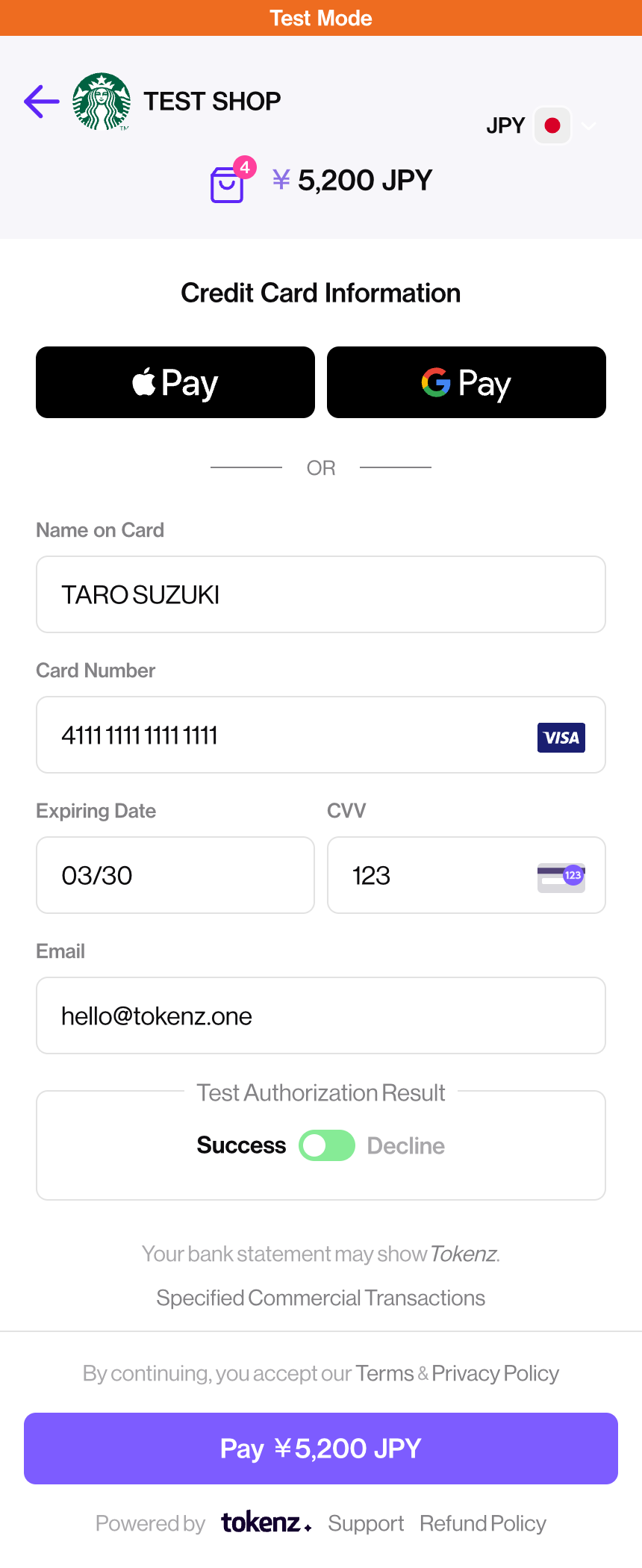
When you're ready to take your integration live, replace your test API keys with live ones. You can't process live payments if your integration uses your test API keys.
Complete a successful test payment
Create a Checkout Session
Create a Checkout Session using our API.
- Use your test API key to create the Checkout Session in test mode.
curl --location 'https://api.tokenz.one/v1/checkoutsession' \
--header 'Content-Type: application/json' \
--header 'Authorization: Bearer {YOUR_TEST_TOKEN}' \
--data '{
"amount": {
"currency": "JPY",
"amount": 5200
},
"itemDetails": [
{
"product": {
"label": "ひとにぎりのエメラルド",
"images": [
"https://images.ctfassets.net/z82qbo7cv7ia/1dWPbk5Qx2M1Qikj6Knyuc/e37b2c26829c0d30793a348ae3adb3b0/fake-pass.webp"
],
"quantity": 3,
"price": {
"currency": "JPY",
"amount": 1200
}
}
},
{
"product": {
"label": "エメラルドの荷車",
"images": [],
"quantity": 1,
"price": {
"currency": "JPY",
"amount": 1600
}
}
}
],
"successUrl": "http://localhost:9000/success",
"pendingUrl": "http://localhost:9000/pending",
"cancelUrl": "http://localhost:9000/cancel",
"customerInfo": {
"emailAddress": "user@email.com"
},
"description": "description",
"reference": "reference",
"theme": "LIGHT"
}'
Complete the Tokenz Checkout
Open the
url
returned by the Create Checkout Session API call.- Select
Card
- Fill out the email address and card details using one of the test cards from below.
- Leave the Test Authorization Result switch as Success. This ensures that your order will be paid successfully.
- Click
Pay
. The payment has been processed successfully, and you will be shown our success page. If you set up webhooks, you will also receive anorder.succeeded
webhook. - When you click
ok
you are redirected to thesuccessUrl
you specified when creating the Checkout Session.
Never use real card details in test mode. Please use a test card from below.
Test Card numbersBrand Number CVC Date Any 3 digits Any future date Any 3 digits Any future date Any 3 digits Any future date Any 3 digits Any future date Any 3 digits Any future date Any 3 digits Any future date Any 4 digits Any future date Any 4 digits Any future date Any 3 digits Any future date Any 3 digits Any future date - Select
![]() | ![]() | ![]() |
Trigger a payment decline
If a user's payment is declined (e.g. their card is declined), we allow the user to try again (e.g. try with a different card). There is no action required from you for a declined payment.
Only fulfill an order if you have received confirmation of a successful payment.
Create a Checkout Session
Create a Checkout Session using our API.
- Use your test API key to create the Checkout Session in test mode.
curl --location 'https://api.tokenz.one/v1/checkoutsession' \
--header 'Content-Type: application/json' \
--header 'Authorization: Bearer {YOUR_TEST_TOKEN}' \
--data '{
"amount": {
"currency": "JPY",
"amount": 5200
},
"itemDetails": [
{
"product": {
"label": "ひとにぎりのエメラルド",
"images": [
"https://images.ctfassets.net/z82qbo7cv7ia/1dWPbk5Qx2M1Qikj6Knyuc/e37b2c26829c0d30793a348ae3adb3b0/fake-pass.webp"
],
"quantity": 3,
"price": {
"currency": "JPY",
"amount": 1200
}
}
},
{
"product": {
"label": "エメラルドの荷車",
"images": [],
"quantity": 1,
"price": {
"currency": "JPY",
"amount": 1600
}
}
}
],
"successUrl": "http://localhost:9000/success",
"pendingUrl": "http://localhost:9000/pending",
"cancelUrl": "http://localhost:9000/cancel",
"customerInfo": {
"emailAddress": "user@email.com"
},
"description": "description",
"reference": "reference"
}'
Complete the Tokenz checkout
Open the
url
returned by the Create Checkout Session API call.- Select
Card
- Fill out the email address and the card details using one of the test cards.
- Change the Test Authorization Result switch to Decline. This ensures that your payment will be declined.
- Click
Pay
. The payment has been declined, and you will be redirected back to the card input page, where you can try the payment again.
- Select
![]() | ![]() |
Complete an asynchronous test payment
You can use convenience store payment to test the payment flow for asynchronous payments. This enables you to test how to deal with delayed payment success notifications.
Create a Checkout Session
Create a Checkout Session using our API.
- Use your test API key to create the Checkout Session in test mode.
curl --location 'https://api.tokenz.one/v1/checkoutsession' \
--header 'Content-Type: application/json' \
--header 'Authorization: Bearer {YOUR_TEST_TOKEN}' \
--data '{
"amount": {
"currency": "JPY",
"amount": 5200
},
"itemDetails": [
{
"product": {
"label": "ひとにぎりのエメラルド",
"images": [
"https://images.ctfassets.net/z82qbo7cv7ia/1dWPbk5Qx2M1Qikj6Knyuc/e37b2c26829c0d30793a348ae3adb3b0/fake-pass.webp"
],
"quantity": 3,
"price": {
"currency": "JPY",
"amount": 1200
}
}
},
{
"product": {
"label": "エメラルドの荷車",
"images": [],
"quantity": 1,
"price": {
"currency": "JPY",
"amount": 1600
}
}
}
],
"successUrl": "http://localhost:9000/success",
"pendingUrl": "http://localhost:9000/pending",
"cancelUrl": "http://localhost:9000/cancel",
"customerInfo": {
"emailAddress": "user@email.com"
},
"description": "description",
"reference": "reference",
"theme": "LIGHT"
}'
Complete the Tokenz checkout
Open the
url
returned by the Create Checkout Session API call.- Make sure
Japan
is selected as country/region in the top right corner - Select
Konbini
as the payment method - Fill out any name, email address, and phone number, and select a convenience store.
- Leave the Test Authorization Result switch as Success. This ensures your order will be paid successfully. Click the
支払う
button to initiate the payment. - The checkout screen displays the instructions for paying at the convenience store.
- Once the payment is made (after 10 seconds, in case of a test payment), a success screen is displayed. If you set up webhooks, you will also receive an
order.succeeded
webhook. - Clicking ok will redirect to the
successUrl
specified when creating the checkout session.
- Make sure
![]() | ![]() |
Do not try to pay for the test payment in a convenience store. Test checkouts are for testing only, and no real payment is accepted or necessary.
Test wallet payments like PayPay or Alipay
During live payments, users are required to log in to their wallets and approve the payment. To allow you to test wallet payments without an account, during test checkout, we only simulate the redirect to wallet providers.
Create a Checkout Session for PayPay payment
Create a Checkout Session using our API.
- Use your test API key to create the Checkout Session in test mode.
- Specify
PAYPAY
as the accepted payment method.
curl --location 'https://api.tokenz.one/v1/checkoutsession' \
--header 'Content-Type: application/json' \
--header 'Authorization: Bearer {YOUR_TEST_TOKEN}' \
--data '{
"amount": {
"currency": "JPY",
"amount": 5200
},
"itemDetails": [
{
"product": {
"label": "ひとにぎりのエメラルド",
"images": [
"https://images.ctfassets.net/z82qbo7cv7ia/1dWPbk5Qx2M1Qikj6Knyuc/e37b2c26829c0d30793a348ae3adb3b0/fake-pass.webp"
],
"quantity": 3,
"price": {
"currency": "JPY",
"amount": 1200
}
}
},
{
"product": {
"label": "エメラルドの荷車",
"images": [],
"quantity": 1,
"price": {
"currency": "JPY",
"amount": 1600
}
}
}
],
"successUrl": "http://localhost:9000/success",
"pendingUrl": "http://localhost:9000/pending",
"cancelUrl": "http://localhost:9000/cancel",
"customerInfo": {
"emailAddress": "user@email.com"
},
"description": "description",
"reference": "reference",
"paymentMethodKinds": [
"PAYPAY"
],
"theme": "LIGHT"
}'
Complete the Tokenz Checkout
Open the
url
returned by the Create Checkout Session API call.- Fill out the email address.
- Set the Test Authorization Result switch to either Success or Decline, depending on your desired outcome.
- Click
Continue with PayPay
, which redirects the user to PayPay to complete the purchase in the case of live payments. In the case of test payments, we only show a placeholder screen, so you don't need an PayPay account to test. - Click
支払う
. Depending on the test result switch, the payment is processed successfully or declined.
![]() | ![]() | ![]() |